- Learning Objective C For Macular Degeneration
- Learning Objectives For Macbeth
- Learning Objective C For Mac Os
Objective-C is the main language for writing software on Apple's Mac OS X computers and iOS devices (iPhone, iPad, and iPod Touch). It's an object-oriented language based on C, but the inclusion of features from Smalltalk give it a unique appearance when compared to similar languages such as C++, C#, and Java.
Product Information. Get up to speed on Cocoa and Objective-C, and start developing applications on the iOS and OS X platforms. If you don't have experience with Apple's developer tools, no problem From object-oriented programming to storing app data in iCloud, the fourth edition of this book covers everything you need to build apps for the iPhone, iPad, and Mac. GNUStep and the Objective-C language (via gcc) are available for many platforms, and you can write your own programs that use Objective-C objects and interact with any C api from Objective-C. Objective-C is a superset of C and so is not really more forgiving than C, but it is arguably more forgiving than C (an almost-superset of C).
This is a short overview of the language and the tools that required to develop software using it. Excellent video resources are available when learning to Program in Objective-C & Xcode, and developers who are particularly interested in mobile apps will enjoy Objective C for iPhone-iPad App Programming.
Getting Started
Apple provides an integrated development environment (IDE) to use when writing software and apps in Objective-C. This IDE is called Xcode and it can only be installed on Apple computers running Mac OS X. However, it is possible to use OS X on a virtual machine in Windows and use Xcode that way.
There are some small differences in the requirements between developing for Mac OS X and iOS, and depending on how you want to distribute your completed projects.
When writing software for OS X or iOS and placing them on Apple's App Stores, you need:
- An Intel Mac running OS X Mavericks (10.9) or Mountain Lion (10.8).
- The latest version of Apple Xcode (5.1.1).
- A Mac Developer Program or iOS Developer Program account.
If you do not have an Apple Developer account, or cannot meet the minimum computer requirements, older versions of Xcode are available for Mac OS X dating back to OS X Panther (10.3) on PowerPC Macs. You will not be able to submit apps to Apple's App Stores, but running an older version of Xcode can be a good way of trying out programming with Objective-C.
Apple has long encouraged application developers to adopt the 64-bit runtime environment, and we've been hearing from customers that 64-bit versions of Office for Mac are desirable to enable larger address spaces, better performance, and new innovative features. All releases of Office for Mac after August 22, 2016 are 64-bit only. 64 bit office for mac.
Downloading and Installing Xcode
The latest version of the Xcode IDE, version 5.1.1, can be downloaded and installed for free from the Mac App Store. Older versions of the program can be found in Apple's Developer Center. You do not need a paid Developer Program account to access the Developer Center, only an Apple ID.
To download Xcode from the Developer Center:
- In your web browser, visit developer.apple.com/xcode/downloads.
- Under Related Tools & Resources, in Additional Tools, click View Downloads >.
- Login with your Apple ID and password.
- Xcode downloads are available in the Developer Tools category.
Not all versions of Xcode support the complete language, or building apps for iOS. In general, if you are planning to distribute your software, you should use the latest version of the IDE. You may not be able to submit your projects for inclusion in the iOS or Mac App Stores if you use any version of Xcode other than the most recent.
After downloading the '.dmg' for a suitable version of the software, you can install most of them on your system in the same way:
- Double-click the downloaded file to 'mount' the file and open it.
- Drag the Xcode icon into your Applications folder.
Learning Objective C For Macular Degeneration
Creating a New Project
You will usually start by selecting one of the predefined application templates. The options available here are slightly different in each version of the IDE.
To try out some of the language features of Objective-C, without worrying about details like user interfaces, you can create a simple command line application:
- On the File menu, click New Project…
- Under Command Line Utility, click Foundation Tool.
- Click Next.
Specify a name for the project and the location where you would like it to be saved, and then click Finish. Xcode will create the necessary code files and configuration settings to give you a jump-start in writing the software.
Objective-C code files are stored with the extension '.m', and there will only be one of these in your project so far. The sample code generated by the application templates tends to vary between different versions of Xcode. However, in the case of a Foundation Tool command line program, it will look something like this:
C programmers will immediately be familiar with most of the code here.
Learning Objectives For Macbeth
This example begins with an #import directive that loads the Foundation library, which contains Objective-C's standard objects and functions.
Next there is the function main(), which is a standard C declaration. In this case, it is called by the operating system when the program is run, and any command line parameters are passed into the array argv. Curly brackets surround the code that is run when that function is called.
It may be obvious already that programming in Objective-C is a mix of writing code in C, and using Smalltalk code when dealing with objects.
Object-Oriented Programming in Objective-C
The code in square brackets on lines 4 and 9 are examples of how Objective-C uses the syntax from Smalltalk for object-oriented programming.
This is line 4:
This assignment statement accomplishes several tasks at once. First it declares a pointer to an NSAutoreleasePool object, and then it creates a new instance of an NSAutoreleasePool and initializes it.
All objects in Objective-C inherit a method called 'alloc' from NSObject. This method is used to allocate memory for the object, and it returns an ID reference not the usable object itself. To call the alloc method, you would use the following statement:
This is the standard syntax for calling a method, without parameters, in Objective-C.
To obtain a usable instance of the object, we first need to call its 'init' method, and this can be done by nesting the allocation statement inside the call to init, as the example below shows:
As a general rule, if you allocate a memory for an object, you must release this memory when you are finished with it.
Line 9 is an example of how to do this by calling the object's release method, which is also inherited from NSObject.
You will spend a lot of time debugging software as you write. NSLog() is defined as a global function in the Foundation library and is not part of any object. It is used for outputting debug messages and is extremely useful when fixing problems in your programs, or when experimenting.
Memory Management in Objective-C
Every time you allocate memory for an object, you must remember to release this memory when you are done. If you do not, the machine may run out of memory and terminate your program unexpectedly. Failing to release memory is also one of the most common reasons that Apple will reject your software or apps when you submit them to the Mac and iOS App Stores.
This process can be tricky for new programmers, and the most common example of this complexity comes with use of one of the most common objects, NSString.
Pages for mac app store. The code above declares a string and then sends it to the debug window. It also demonstrates how some objects can be created without specifically calling the alloc and init methods.
Learning Objective C For Mac Os
However, the call to release message terminates the program with an error.
Generally speaking, unless you use the method alloc to allocate memory, you will not need to release it. If you are in any doubt, you can always try calling the release method, and removing it if it causes an error.
If you are writing for Mac OS X Lion (10.7) or IOS 5 and later versions of those operating systems, you may be relieved to learn that an extension known as 'Automatic Reference Counting' (ARC) was added to help with memory management. This removes some of the confusion surrounding when you should, and should not, release objects. However, it is in the nature of Objective-C programming that you must pay attention to memory management – disabling ARC and releasing memory yourself is extremely educational, and many developers enjoy the extra control that this gives them.
It's Mostly Just Practice
As this has been just a taster of the language, it may seem like a large jump from where you are now, to actually writing software in Objective-C. Why not learn by building 14 apps, or take a project-based approach to writing software in Xcode?
- Basic Objective-C
- Advanced Objective-C
- Objective-C Useful Resources
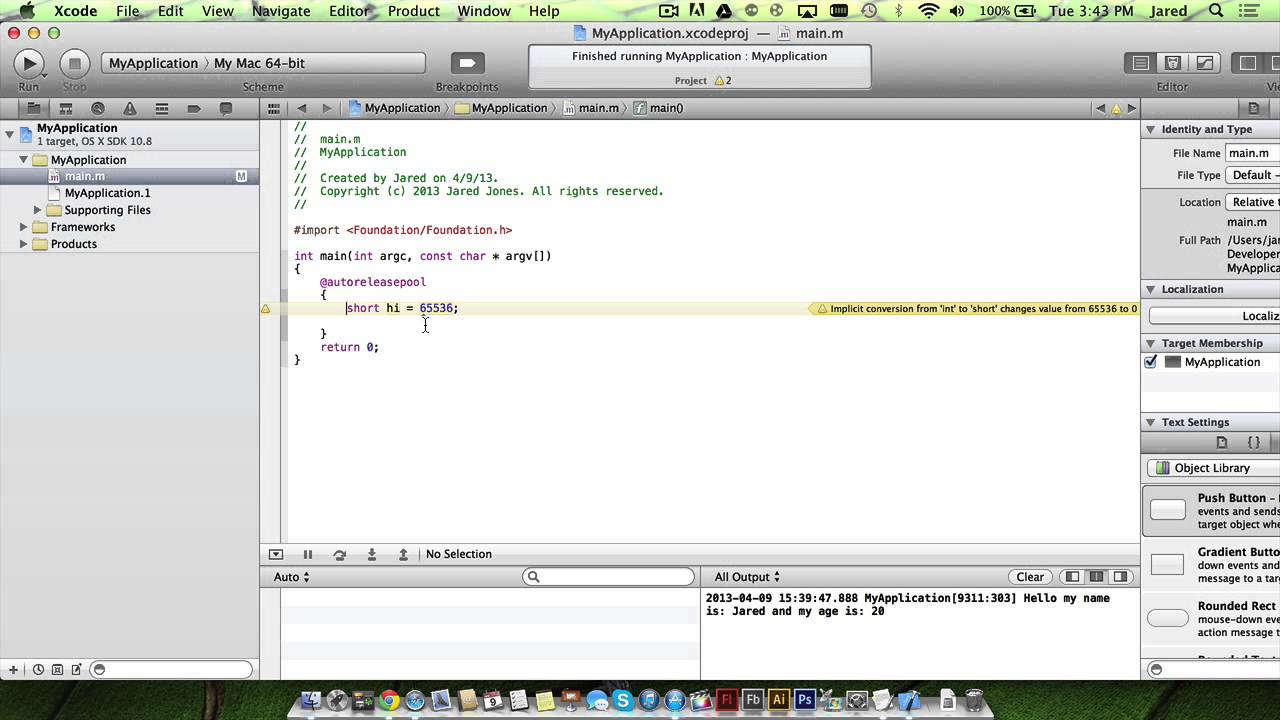
- Selected Reading
Objective-C is general-purpose language that is developed on top of C Programming language by adding features of Small Talk programming language making it an object-oriented language. It is primarily used in developing iOS and Mac OS X operating systems as well as its applications.
Initially, Objective-C was developed by NeXT for its NeXTSTEP OS from whom it was taken over by Apple for its iOS and Mac OS X.
Object-Oriented Programming
Objective-C fully supports object-oriented programming, including the four pillars of object-oriented development −
- Encapsulation
- Data hiding
- Inheritance
- Polymorphism
Example Code
Foundation Framework
Foundation Framework provides large set of features and they are listed below.
It includes a list of extended datatypes like NSArray, NSDictionary, NSSet and so on.
It consists of a rich set of functions manipulating files, strings, etc.
It provides features for URL handling, utilities like date formatting, data handling, error handling, etc.
Learning Objective-C
The most important thing to do when learning Objective-C is to focus on concepts and not get lost in language technical details.
The purpose of learning a programming language is to become a better programmer; that is, to become more effective at designing and implementing new systems and at maintaining old ones.
Use of Objective-C
Objective-C, as mentioned earlier, is used in iOS and Mac OS X. It has large base of iOS users and largely increasing Mac OS X users. And since Apple focuses on quality first and its wonderful for those who started learning Objective-C.